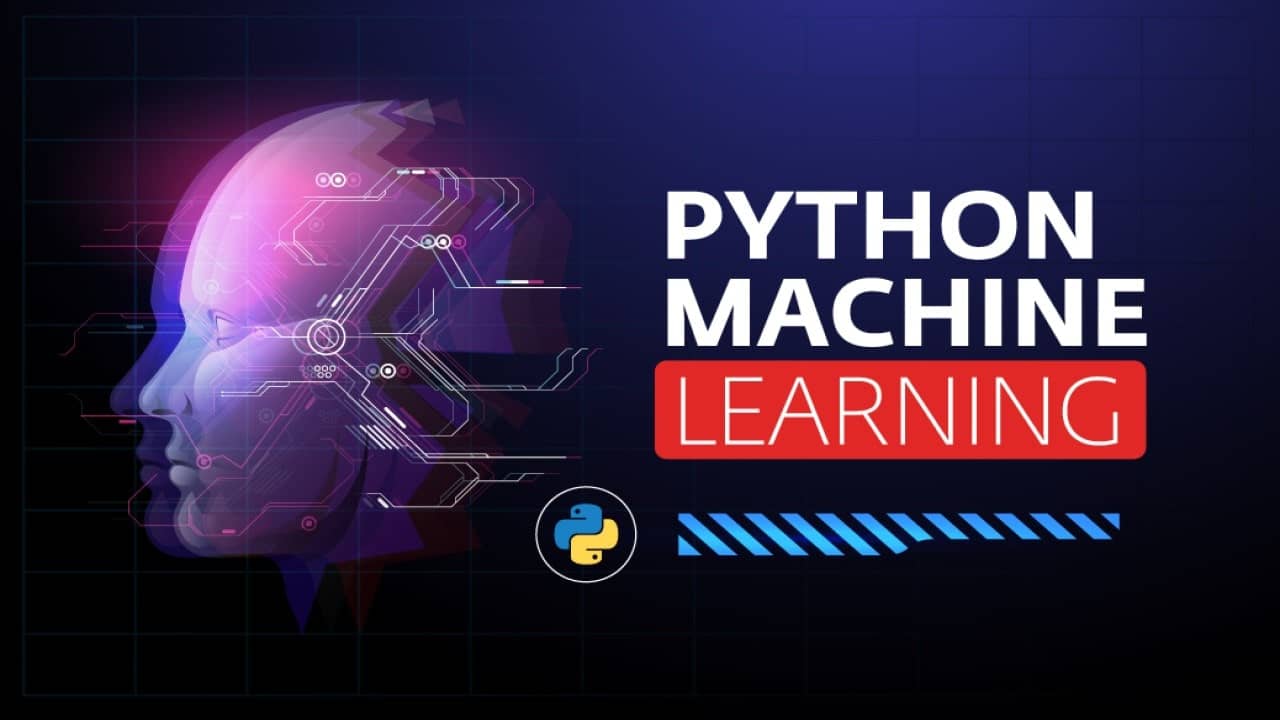
Real-Life Applications of Top Machine Learning Libraries in Python
Introduction:
Machine learning has transformed industries worldwide, enabling data-driven decision-making and automating complex tasks. In this article, we’ll explore practical applications of popular Python machine learning libraries—Scikit-learn, TensorFlow, PyTorch, and Keras—across different domains. Through concrete examples and code snippets, we’ll showcase how these libraries are leveraged to address real-world challenges effectively.
- Predictive Maintenance with Scikit-learn:
Use Case:
Predictive maintenance is crucial for industries like manufacturing, where equipment failure can lead to costly downtime. Scikit-learn facilitates the development of predictive maintenance models using historical sensor data and maintenance logs.
Explanation with code:
# Load data
import pandas as pd
data = pd.read_csv('sensor_data.csv')
# Preprocess data
X = data.drop(columns=['target'])
y = data['target']
# Train predictive model
from sklearn.ensemble import RandomForestClassifier
model = RandomForestClassifier()
model.fit(X, y)
# Predict equipment failure
new_data = pd.read_csv('new_sensor_data.csv')
predictions = model.predict(new_data)
Explanation of Predictive Maintenance Code Snippet:
– Load and Preprocess Data: We load historical sensor data from a CSV file, which contains readings from various sensors installed on the equipment. We split the data into features (sensor readings) and labels (maintenance events).
– Train Predictive Model: Using Scikit-learn’s RandomForestClassifier, we train a machine learning model on the historical sensor data. This model learns to identify patterns in sensor readings indicative of potential equipment failures.
– Predict Equipment Failure: Once the model is trained, we can use it to predict equipment failures based on new sensor data. By inputting recent sensor readings into the model, we can anticipate maintenance needs and take proactive measures to prevent downtime.
Example Data CSV File (sensor_data.csv):
sensor_1,sensor_2,sensor_3,...,target
23.5, 45.1, 67.8,...,1
18.2, 41.7, 69.3,...,0
..., ..., ...,...,...
New Sensor Data CSV and Its Explanation:
Explanation:
The new_sensor_data.csv file contains the latest sensor readings from the equipment. It includes various sensor measurements such as temperature, pressure, and vibration levels. By inputting this data into our predictive maintenance model developed with Scikit-learn, we can predict potential equipment failures and take proactive maintenance actions to prevent downtime.
Example Data CSV File (new_sensor_data.csv):
temperature, pressure, vibration, target
25.6, 101.3, 0.012, 0
30.1, 99.8, 0.018, 1
........, ..., ..., ...
- Image Classification with TensorFlow:
Use Case:
Image classification is vital in healthcare, retail, and autonomous vehicles. TensorFlow’s robust capabilities make it suitable for building and training convolutional neural networks (CNNs) to classify images accurately.
Explanation with code:
# Build CNN model
import tensorflow as tf
model = tf.keras.Sequential([
tf.keras.layers.Conv2D(32, (3, 3), activation='relu', input_shape=(28, 28, 1)),
tf.keras.layers.MaxPooling2D((2, 2)),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(10, activation='softmax')
])
# Compile and train model
model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy'])
model.fit(train_images, train_labels, epochs=10, validation_data=(test_images, test_labels))
Explanation of Image Classification Code Snippet:
– Build CNN Model: We create a convolutional neural network (CNN) model using TensorFlow’s Sequential API. CNNs are specialized neural networks designed to process and classify images. They consist of multiple layers, including convolutional layers for feature extraction and pooling layers for spatial downsampling.
– Compile and Train Model: After building the CNN model, we compile it with an optimizer and loss function, specifying metrics for evaluation. Then, we train the model on a dataset of labeled images, allowing it to learn patterns and associations between image features and their corresponding labels.
Explanation of ReLU and Sigmoid Activation Functions:
– ReLU (Rectified Linear Unit): ReLU is an activation function commonly used in neural networks. It introduces nonlinearity by outputting the input value if it is positive and zero otherwise. In layman’s terms, ReLU allows the neural network to learn complex patterns and relationships by enabling it to model nonlinearities in the data. It is computationally efficient and helps prevent the vanishing gradient problem during training.
– Sigmoid Activation Function: The sigmoid function squashes the input value to a range between 0 and 1. It is often used in binary classification tasks where the output represents the probability of a particular class. In layman’s terms, the sigmoid function converts the raw output of the neural network into probabilities, making it suitable for tasks where we need to make binary decisions or predictions.
- Sentiment Analysis with PyTorch:
Use Case:
Sentiment analysis is used to determine the sentiment or opinion expressed in a piece of text. It finds applications in social media monitoring, customer feedback analysis, and market research. PyTorch’s flexibility and deep learning capabilities make it suitable for building and training sentiment analysis models.
Explanation with code:
# Sample data for sentiment analysis
data = [
("I love this movie", 1),
("This movie is terrible", 0),
("The acting was great", 1),
("The plot was boring", 0)
]
# Tokenize text data and create vocabulary
word_to_idx = {}
for sentence, _ in data:
for word in sentence.split():
if word not in word_to_idx:
word_to_idx[word] = len(word_to_idx)
# Convert text data to numerical format
numerical_data = []
for sentence, label in data:
numerical_sentence = [word_to_idx[word] for word in sentence.split()]
numerical_data.append((numerical_sentence, label))
# Define PyTorch dataset and dataloader
import torch
from torch.utils.data import Dataset, DataLoader
class SentimentDataset(Dataset):
def __init__(self, data):
self.data = data
def __len__(self):
return len(self.data)
def __getitem__(self, index):
return self.data[index]
dataset = SentimentDataset(numerical_data)
dataloader = DataLoader(dataset, batch_size=2, shuffle=True)
# Define LSTM model for sentiment analysis
import torch.nn as nn
class SentimentLSTM(nn.Module):
def __init__(self, vocab_size, embedding_dim, hidden_size, output_size):
super(SentimentLSTM, self).__init__()
self.embedding = nn.Embedding(vocab_size, embedding_dim)
self.lstm = nn.LSTM(embedding_dim, hidden_size)
self.fc = nn.Linear(hidden_size, output_size)
def forward(self, x):
embedded = self.embedding(x)
output, _ = self.lstm(embedded)
output = self.fc(output[:, -1, :])
return output
# Define hyperparameters
vocab_size = len(word_to_idx)
embedding_dim = 100
hidden_size = 128
output_size = 2
learning_rate = 0.001
num_epochs = 10
# Instantiate model, loss function, and optimizer
model = SentimentLSTM(vocab_size, embedding_dim, hidden_size, output_size)
criterion = nn.CrossEntropyLoss()
optimizer = torch.optim.Adam(model.parameters(), lr=learning_rate)
# Training loop
for epoch in range(num_epochs):
for inputs, labels in dataloader:
optimizer.zero_grad()
outputs = model(inputs)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
Explanation of Sentiment Analysis Code Snippet:
– Sample Data for Sentiment Analysis: We start with sample data containing text sentences and their corresponding sentiment labels (1 for positive sentiment, 0 for negative sentiment).
– Tokenize Text Data and Create Vocabulary: We tokenize the text data and create a vocabulary mapping each unique word to a numerical index.
– Convert Text Data to Numerical Format: We convert the text data into numerical format by replacing each word with its corresponding index in the vocabulary.
– Define PyTorch Dataset and DataLoader: We define a custom PyTorch dataset and dataloader to efficiently handle the numerical data during training.
– Define LSTM Model for Sentiment Analysis: We define a Long Short-Term Memory (LSTM) model using PyTorch’s nn-module class. The LSTM model is capable of understanding the sequential nature of text data and capturing long-term dependencies.
– Instantiate Model, Loss Function, and Optimizer: We instantiate the LSTM model, define the loss function (CrossEntropyLoss), and specify the optimizer (Adam) for training the model.
- Keras for Fast Experimentation:
Use Case:
Keras simplifies deep learning model building and experimentation. It provides a high-level API for building and training neural networks, enabling rapid prototyping and experimentation.
Explanation with code:
# Python Keras – Environment Setup
conda install -c anaconda keras-gpu
# Build and train Keras model
import keras
from keras.models import Sequential
from keras.layers import Dense
# Define model
model = Sequential([
Dense(64, activation='relu', input_dim=100),
Dense(64, activation='relu'),
Dense(1, activation='sigmoid')
])
# Compile model
model.compile(optimizer='adam',
loss='binary_crossentropy',
metrics=['accuracy'])
# Train model
model.fit(X_train, y_train, epochs=10, batch_size=32, validation_data=(X_val, y_val))
Explanation of Keras Code Snippet:
– Build Keras Model: We create a sequential model using Keras, specifying the layers and activation functions. The model consists of densely connected layers with ReLU activation functions and a final output layer with a sigmoid activation function.
– Compile Model: We compile the model with an optimizer, loss function, and evaluation metrics. Here, we use the Adam optimizer and binary cross-entropy loss for binary classification tasks.
– Train Model: We train the model on training data, specifying the number of epochs, batch size, and validation data for evaluation during training.
Explanation of Neural Networks:
Neural networks are a class of machine learning models inspired by the structure and function of the human brain. They consist of interconnected nodes, called neurons, organized into layers. Neural networks are capable of learning complex patterns and relationships from data through a process known as training.
– Input Layer: The input layer receives the initial data or features to be processed by the neural network.
– Hidden Layers: Hidden layers are intermediate layers between the input and output layers. They perform nonlinear transformations on the input data, enabling the neural network to learn complex representations and patterns.
– Output Layer: The output layer produces the final predictions or outputs of the neural network based on the processed input data.
– Activation Functions: Activation functions introduce nonlinearity into the neural network, allowing it to model complex relationships between inputs and outputs. Common activation functions include ReLU (Rectified Linear Unit) and sigmoid.
– Weights and Biases: Neural networks learn from data by adjusting the weights and biases associated with each neuron. These parameters control the strength of connections between neurons and determine the output of the network for a given input.
Conclusion:
Machine learning libraries in Python empower developers and data scientists to solve real-world problems across diverse domains. By leveraging libraries like Scikit-learn, TensorFlow, PyTorch, and Keras, practitioners can drive innovation and make meaningful impacts in their respective fields. Whether it’s predicting equipment failures, classifying images, analyzing text data, or prototyping deep learning models, Python’s rich ecosystem of machine learning libraries provides the tools and capabilities needed to build sophisticated solutions.